Using Rcpp in Xcode
Introduction
In this tutorial, we’ll look at how you can configure Xcode to work with and debug Rcpp objects and methods.
Why?
I’m interested in building fast machine learning models with C++ and exposing those models to R, much like ranger and xgboost. Rcpp makes it easy to pass objects between R and C++. However, the only way to debug Rcpp code within RStudio that I’m aware of is to litter the code with print statements. On the other hand, Xcode is a fantastic IDE (for mac os) to build C++ applications. It comes equipped with a very handy debugging tool and I’d like to use it to step through and debug my Rcpp code.
Before we get started, let’s take a look at the end result in action.
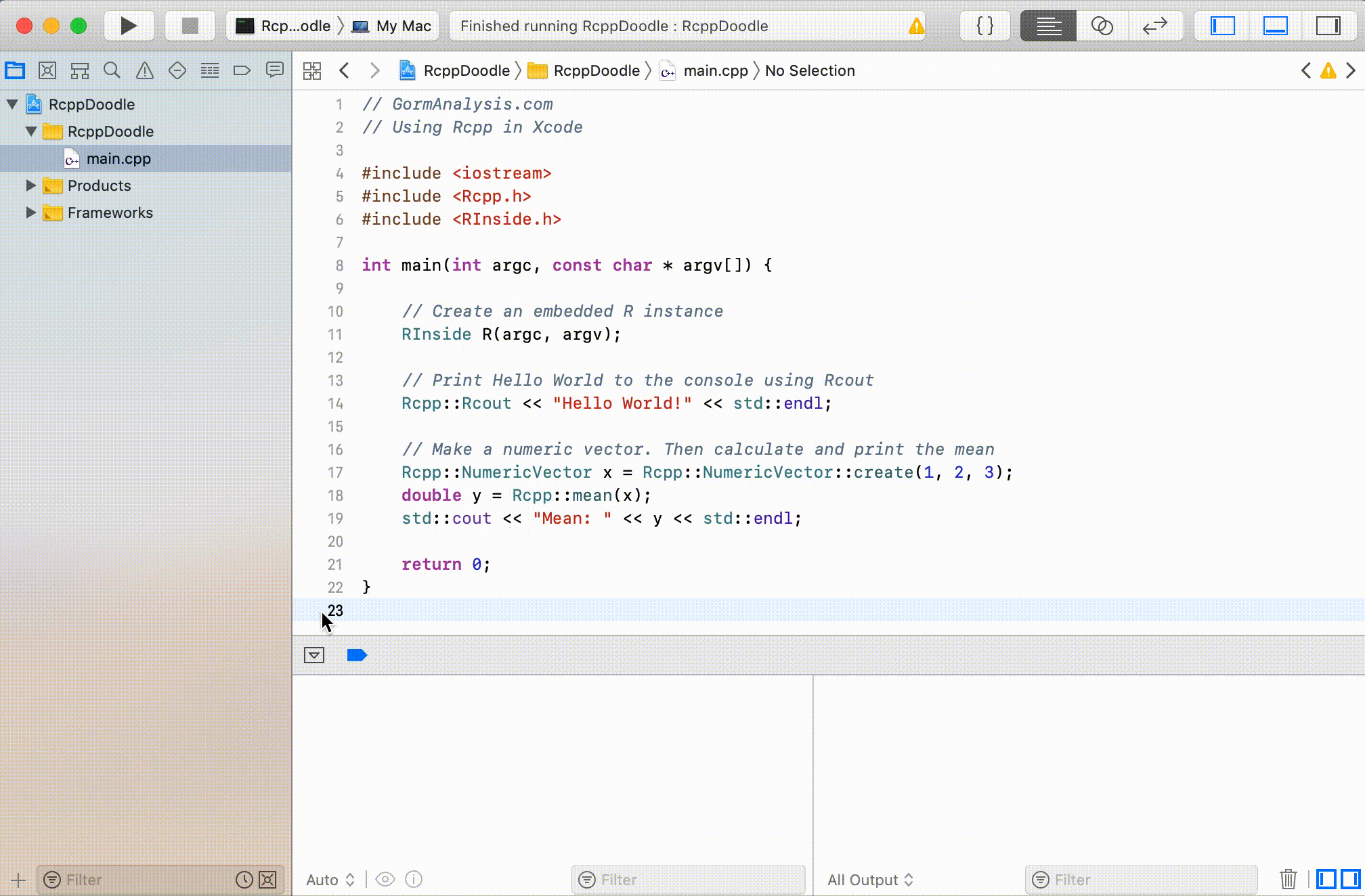
Setup
Firstly, I’ll assume you have R, Rcpp, and Xcode installed and working.
- From R, install RInside with
install.packages("RInside")
- Add necessary R header files to the Xcode Header Search Paths. For me, these paths are
- /Library/Frameworks/R.framework/Headers/
- /Library/Frameworks/R.framework/Versions/3.5/Resources/library/RInside/include/
- /Library/Frameworks/R.framework/Versions/3.5/Resources/library/Rcpp/include/
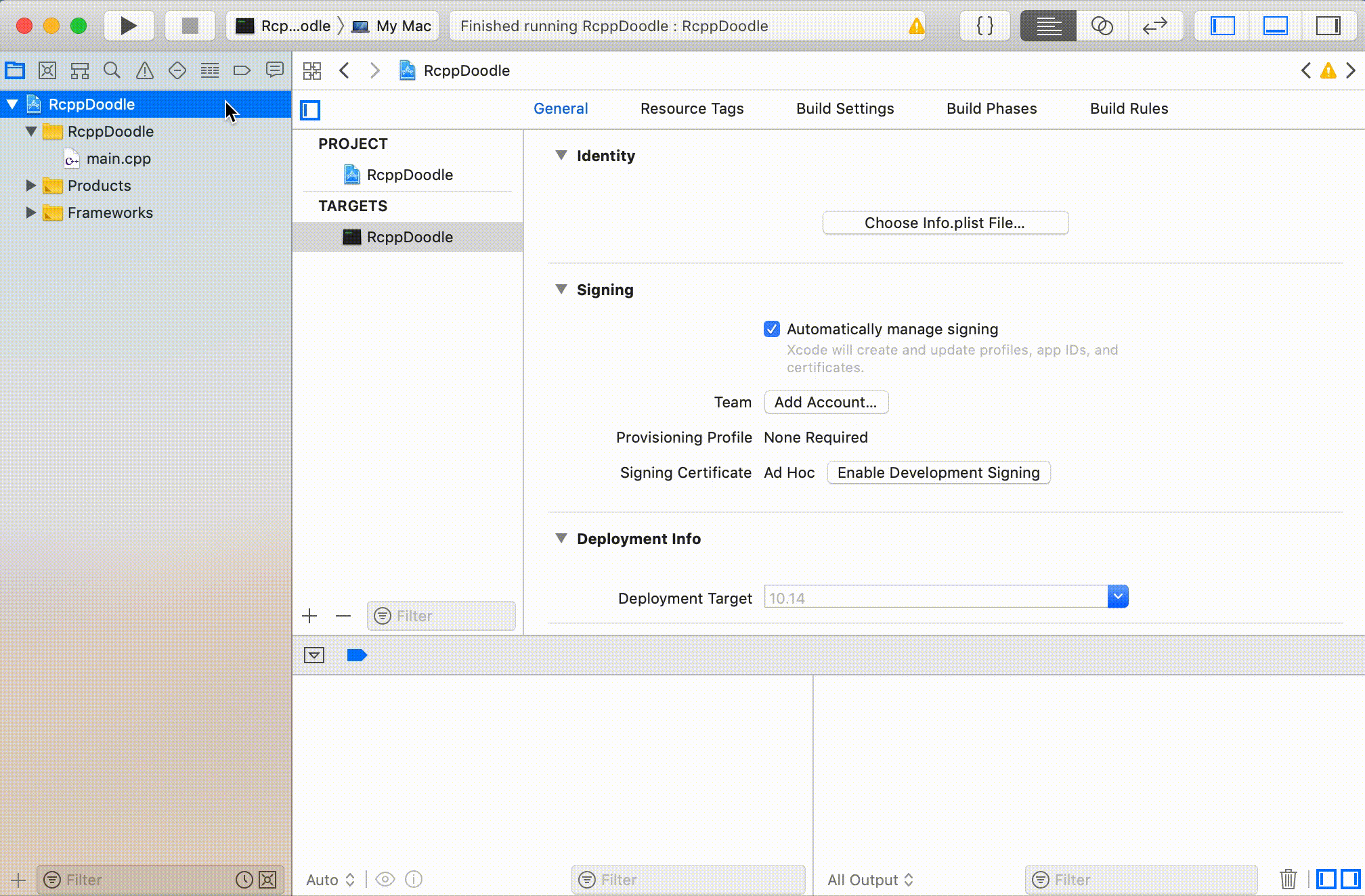
- Add the R and RInside dynamic libraries to your Xcode project. For me, these are located in
- /Library/Frameworks/R.framework/Versions/3.5/Resources/lib/libR.dylib
- /Library/Frameworks/R.framework/Versions/3.5/Resources/library/RInside/lib/libRInside.dylib
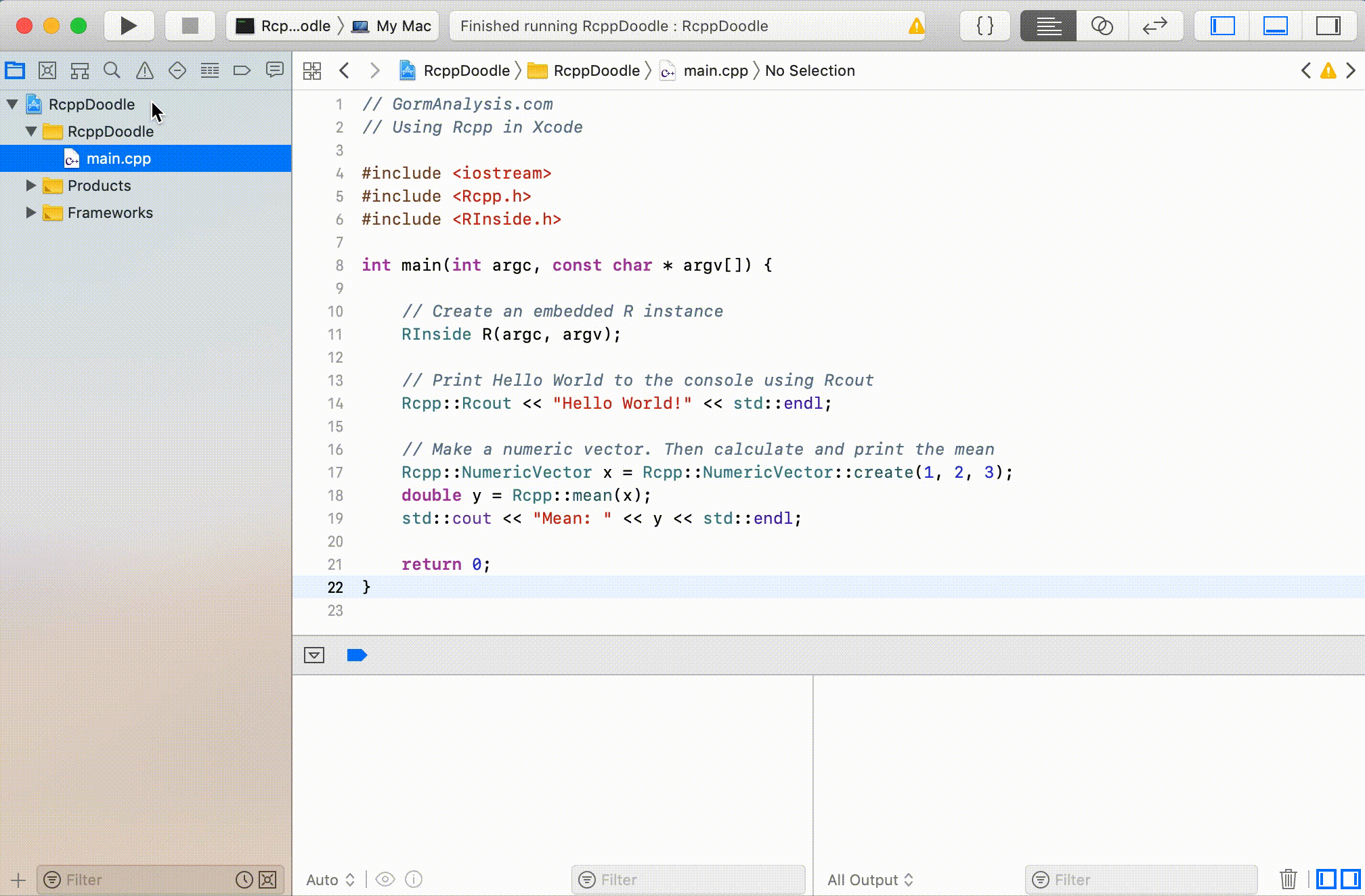
#include <Rcpp.h>
and#include <RInside.h>
somwhere in your C++ project- Inside main, create an embedded R instance with
RInside R(argc, argv);
- Give it a go!
#include <iostream>
#include <Rcpp.h>
#include <RInside.h>
int main(int argc, const char * argv[]) {
// Create an embedded R instance
RInside R(argc, argv);
// Print Hello World to the console using Rcout
Rcpp::Rcout << "Hello World!" << std::endl;
// Make a numeric vector. Then calculate and print the mean
Rcpp::NumericVector x = Rcpp::NumericVector::create(1, 2, 3);
double y = Rcpp::mean(x);
std::cout << "Mean: " << y << std::endl;
return 0;
}
Special Thanks
I owe special thanks to Brian Hall for his article Linking Xcode, C++ and R to create plots. This article is basically a re-write of his. And of course thanks to Dirk Eddelbuettel for making Rcpp and RInside.