An R User's Guide To Setting Up Python
In this guide I’ll cover how I set up Python with a few tips and tricks to make it an easier transition from R and RStudio.
Anaconda
We’ll be using the Anaconda distribution to install Python. AFAIK, the main reason Anaconda exists is because it allows you to have multiple instances of Python installed and potentially running at the same time. If you do freelance work like myself, this can be useful if client ABC uses Python 3.3 and client DEF uses Python 3.7. With Anaconda, I can have both versions running on my Mac, and I can switch between the two with ease. I can also remove Anaconda-installed Python environments without worrying about deleting important system files.
Download And Install
This guide is likely to get outdated fast, so browse and install the most up-to-date Anaconda Distribution here. For me, this’ll be “Python 3.7 version” on macOS.
After downloading, go through the installation. I keep the default settings unchanged. Once this is done, I recommend finding where anaconda was installed on your system. In my case, it was installed at /anaconda3
. (This is important if you need to modify your $PATH or if you want to remove the distribution in the future.)
Basic Use
To check that things are working, pull up a terminal window/command prompt and run conda --version
. (I get “conda 4.7.5”)
Create a new environment
Here we create a new python environment called env1 and a second one called env2.
conda create --name env1
conda create --name env2
Check what environments exist
conda info --envs
Activate a specific environment
conda activate env1
Install a new package into the active environment
conda install scipy
or
conda install pip; pip install scipy
Check what packages are installed in the active environment
conda list
Running Python inside the active environment
In order to run a snippet of python, just execute python
(after having activated a conda environment with something like conda activate env1
). Then you can do
print("hello world")
# hello world
quit()
Deactivate the currently active environment
conda deactivate
PyCharm
PyCharm is like the RStudio of Python.
Download and Install
Download and install the free, opensource Community version of PyCharm. As with Anaconda, I use PyCharm’s default settings.
Once installed, you’ll want to create a new project and choose your Python interpreter. Here, I create a new project called my-project and choose the env1 python environment I created earlier with Anaconda.
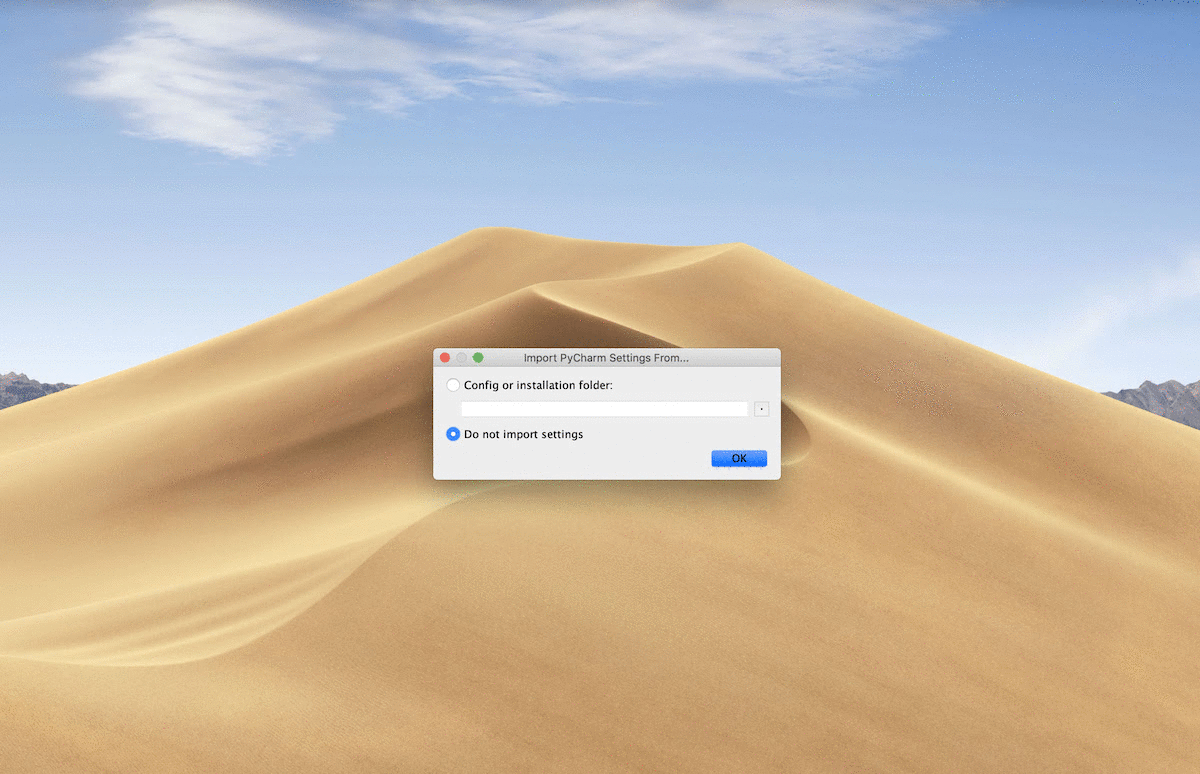
Create a new Python file
Create a new python file by right-clicking on the my-project folder in the project explorer and then selecting New > Python File. I’ll call this file hello_world.py
Executing Code
There are a few ways to execute code.
Python Console
Click on the on tab labeled Python Console at the bottom of the window. Here you run python code just as you would run R code directly in the RStudio Console window.
Clear the console
- Right-click on the console > Clear All
- Preferred Method: Create a keyboard shortcut for this to match RStudio’s Clear Console command (ctrl+l)
Python Script
You can run code from a python script just as in RStudio as well. To do this,
- Click the Add Configuration button near the top of the window.
- Click the
+
symbol > Python to add a new Python configuration - Choose the current script’s path
- Check the box that says “Run with Python Console”
- Click Apply and then OK
Run entire script
- Click the green run button near the top right
Run a single line of code
- Right-click on the line > Execute Line in Console
- Use the keyboard shortcut option+shift+e
- Preferred Method Create a keyboard shortcut for this to match RStudio’s execute command (cmd+return)
Run a highlighted snippet of code
- Right-click highlighted code > Execute Selection in Console
- Use the keyboard shortcut option+shift+e
- Preferred Method: Create a keyboard shortcut for this to match RStudio’s execute command (cmd+return)
Comment a line of code
- Click on a line of code > cmd+/
- Preferred Method: Create a keyboard shortcut for this to match RStudio’s execute command (cmd+shift+c)
Comment a block of code
- Highlight a block of code > cmd+/
- Preferred Method: Create a keyboard shortcut for this to match RStudio’s execute command (cmd+shift+c)
Managing Packages
We’ve already discussed how to install new Python packages via Terminal/Command Prompt. The PyCharm GUI makes this easier. For example, click PyCharm > Preferences > Project > Project Interpreter
From here you can view, add, or remove existing packages in the active python environment.